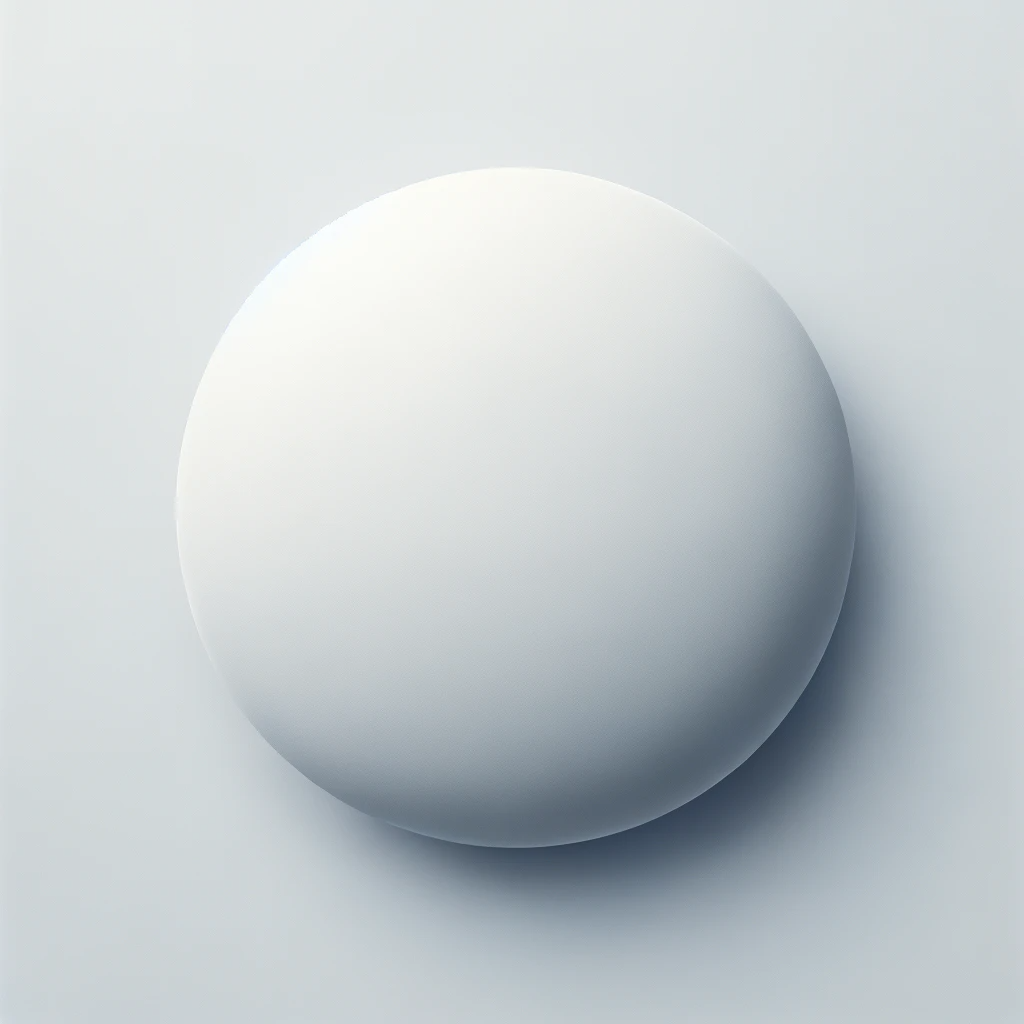
You don’t have to dig a big hole in your yard to cut a piece of plastic PVC pipe that’s buried in the ground. Watch this video to find out how to cut PVC pipe using nothing more th...Does Python have a string contains substring method? 99% of use cases will be covered using the keyword, in, which returns True or False: 'substring' in any_string For the use case of getting the index, use str.find (which returns -1 on failure, and has optional positional arguments):. start = 0 stop = len(any_string) any_string.find('substring', start, …File "main.py", line 1. my_string = 'It's a lovely day!' ^ SyntaxError: invalid syntax. ... Python has a number of built-in string methods that manipulate strings. However, when these methods are called, the original string will not be changed, so any modifications will need to be saved to a new variable. A few useful built-in string methods ...pandas.Series.str. #. Series.str() [source] #. Vectorized string functions for Series and Index. NAs stay NA unless handled otherwise by a particular method. Patterned after Python’s string methods, with some inspiration from R’s stringr package.index (substring, start, end) Return the index of first occurrence in the string where substring is found. Raises an exception if the substring is not present in the given string. If we do not give start index and end index then searching starts from index 0 and ends at length of the string. >>> str ='banana'. >>> str.index('an') 1.A String is a data structure in Python that represents a sequence of characters. It is an immutable data type, meaning that once you have created a string, you cannot change it. Strings are used widely in many different applications, such as storing and manipulating text data, representing names, addresses, and other types of data that can …Reverse string in Python using stack. An empty stack is created. One by one character of the string is pushed to the stack. One by one all characters from the stack are popped and put back to a string. Time complexity: O(n) Auxiliary Space: O(n) Implementation:Convert to title case: str.title () The title () method converts the first character of each word in the string to uppercase and the rest to lowercase. s_org = 'pYThon proGramminG laNguAge' print(s_org.title()) # Python Programming Language. source: str_upper_lower.py.Using variables in SQL statements can be tricky, but they can give you the flexibility needed to reuse a single SQL statement to query different data. In Visual Basic for Applicati...May 11, 2023 ... Python Programming: String Methods in Python (Part 3) Topics discussed: 1. split() Method for Strings in Python. 2. rsplit() Method for ...The startswith() method returns True if the string starts with the specified value and False otherwise. The endswith() method works the same way but with the end of a string. These methods take three arguments, the first of which is required while the latter two are optional. The first argument is the value you are looking to see if the string ...Python String Methods; Python List Methods; Python Set Methods; Python Dictionary Methods; Python slice() function. The slice() method returns a portion of an iterable as an object of the slice class based on the specified range. It can be used with string, list, tuple, set, bytes, or range objects or custom class object that implements sequence methods …Python documentation strings (or docstrings) provide a convenient way of associating documentation with Python modules, functions, classes, and methods. It’s specified in source code that is used, like a comment, to document a specific segment of code. Unlike conventional source code comments, the docstring should describe what …Python String Methods. Python string class has a set of methods, which we can use on String objects. In this tutorial, we shall go through each of the method, what it does, … Python has a set of built-in methods that you can use on dictionaries. Method. Description. clear () Removes all the elements from the dictionary. copy () Returns a copy of the dictionary. fromkeys () Returns a dictionary with the specified keys and value. Nov 19, 2019 ... In this video I talk about the different string methods you can use in Python. Need one-on-one help with your project?Apr 27, 2023 · Python offers many ways to check whether a String contains other substrings or not. Some of them are given below: Using the find () Method. Using the in Operator. Using the index () Method. Using the regular expression. Using the string __contains__ () The ‘in’ operator, find (), and index () methods are frequently used to check for a ... The TouchStart string trimmer from Ryobi features an easy to use 12-volt, battery powered, electric starting system. Expert Advice On Improving Your Home Videos Latest View All Gui...In Python, “strip” is a method that eliminates specific characters from the beginning and the end of a string. By default, it removes any white space characters, such as spaces, ta... W3Schools offers free online tutorials, references and exercises in all the major languages of the web. Covering popular subjects like HTML, CSS, JavaScript, Python, SQL, Java, and many, many more. Serial numbers are the unique string of numbers and/or letters that are stamped on goods of value. They have several purposes, one which makes your item identifiable to the manufac...Python has a set of built-in methods that you can use on lists/arrays. Add the elements of a list (or any iterable), to the end of the current list. Returns the index of the first element with the specified value. Note: Python does not have built-in support for Arrays, but Python Lists can be used instead.String operations #. Return element-wise string concatenation for two arrays of str or unicode. Return (a * i), that is string multiple concatenation, element-wise. Return (a % i), that is pre-Python 2.6 string formatting (interpolation), element-wise for a pair of array_likes of str or unicode.As with any dairy-based product, string cheese should be refrigerated until it is ready to be eaten. String cheese is safe to eat for up to 2 hours before it should be refrigerated...Python has quite a few methods that string objects can call to perform frequency occurring task (related to string). For example, if you want to capitalize the ...String operations #. Return element-wise string concatenation for two arrays of str or unicode. Return (a * i), that is string multiple concatenation, element-wise. Return (a % i), that is pre-Python 2.6 string formatting (interpolation), element-wise for a pair of array_likes of str or unicode.File "main.py", line 1. my_string = 'It's a lovely day!' ^ SyntaxError: invalid syntax. ... Python has a number of built-in string methods that manipulate strings. However, when these methods are called, the original string will not be changed, so any modifications will need to be saved to a new variable. A few useful built-in string methods ...We would like to show you a description here but the site won’t allow us.Lists are one of 4 built-in data types in Python used to store collections of data, the other 3 are Tuple, Set, and Dictionary, all with different qualities and usage. Lists are created using square brackets: Example. Create a List: thislist = ["apple", "banana", "cherry"] print (thislist) Try it Yourself ».Python String Methods. Python, like the set, list, and dictionary class methods, has a plethora of built-in functions for manipulating strings. Python String Methods are a set of built-in methods that you can use on strings for data handling and manipulation.On this article, we’ll cowl helpful Python string strategies for manipulating string (str) objects — corresponding to becoming a member of, splitting and capitalizing. Every methodology described on this article will embrace a proof with a related instance. We’ll additionally finish with slightly problem you need to attempt to assess how a lot … 45. Python String zfill () Method. The zfill () method returns a string with the digits filled in from the left with zeros. For example, if the length of the string is 5 and we zfill () it with a length of 10, then the string will be padded with 5 zeros from the left. Add a comment. 9. def kill_char(string, n): # n = position of which character you want to remove. begin = string[:n] # from beginning to n (n not included) end = string[n+1:] # n+1 through end of string. return begin + end. print kill_char("EXAMPLE", 3) # "M" removed. I have seen this somewhere here. Share.File "main.py", line 1. my_string = 'It's a lovely day!' ^ SyntaxError: invalid syntax. ... Python has a number of built-in string methods that manipulate strings. However, when these methods are called, the original string will not be changed, so any modifications will need to be saved to a new variable. A few useful built-in string methods ...The simplest way to append to a string in python is to use the + (plus) operator. Instead of functioning as a mathematical addition, in Python the + operator is used to concatenate strings together. This means that you can use it to append one string to another. Here’s an example of the + operator in practice:We would like to show you a description here but the site won’t allow us.We would like to show you a description here but the site won’t allow us.join() Parameters. The join() method takes an iterable (objects capable of returning its members one at a time) as its parameter.. Some of the example of iterables are: Native data types - List, Tuple, String, Dictionary and Set. File objects and objects you define with an __iter__() or __getitem()__ method.; Note: The join() method provides a flexible way to …In this tutorial, you'll learn how to concatenate strings in Python. You'll use different tools and techniques for string concatenation, including the concatenation operators and the .join() method. You'll also explore other tools that can also be handy for string concatenation in Python. ... You can call the .join() method on a string object that will work as a separator in …Just a simple contribution. If the class that we need to instance is in the same file, we can use something like this: # Get class from globals and create an instance. m = globals()['our_class']() # Get the function (from the instance) that we need to call. func = getattr(m, 'function_name') # Call it.String indexing in Python is zero-based: the first character in the string has index 0, the next has index 1, and so on. The index of the last character will be the length of the string minus one. For example, a schematic diagram of the indices of the string 'foobar' would look like this: String Indices. W3Schools offers free online tutorials, references and exercises in all the major languages of the web. Covering popular subjects like HTML, CSS, JavaScript, Python, SQL, Java, and many, many more. Python has a set of built-in methods that you can use on dictionaries. Method. Description. clear () Removes all the elements from the dictionary. copy () Returns a copy of the dictionary. fromkeys () Returns a dictionary with the specified keys and value. Strings come bundled with special functions— they’re called string methods—that you can use to work with and manipulate strings. There are numerous string methods …Python strings have a lot of functionality that you can leverage in your scripts. This includes common text operations like searching and replacing text, removing whitespace, or counting characters and words. Collectively, these functions are called Python string methods. In this article, we’ll go through an overview of the main string ... W3Schools offers free online tutorials, references and exercises in all the major languages of the web. Covering popular subjects like HTML, CSS, JavaScript, Python, SQL, Java, and many, many more. String count () function is an inbuilt function in Python programming language that returns the number of occurrences of a substring in the given string. Unmute. ×. It is a very useful string function that can be used for string analysis. Note: string count () function is case sensitive, meaning it will treat ‘a’ and ‘A’ different.The simplest way to append to a string in python is to use the + (plus) operator. Instead of functioning as a mathematical addition, in Python the + operator is used to concatenate strings together. This means that you can use it to append one string to another. Here’s an example of the + operator in practice:Converting Between Strings and Lists. Methods in this group convert between a string and some composite data type by either pasting objects together to make a string, or by breaking a string up into pieces. These methods operate on or return iterables, the general Python term for a sequential collection of objects. You will explore the inner ... W3Schools offers free online tutorials, references and exercises in all the major languages of the web. Covering popular subjects like HTML, CSS, JavaScript, Python, SQL, Java, and many, many more. W3Schools offers free online tutorials, references and exercises in all the major languages of the web. Covering popular subjects like HTML, CSS, JavaScript, Python, SQL, Java, and many, many more. W3Schools offers free online tutorials, references and exercises in all the major languages of the web. Covering popular subjects like HTML, CSS, JavaScript, Python, SQL, Java, and many, many more. 9.5. String Methods¶ ; tess.right(90) when we wanted the turtle object ; tess to perform the ; right method to turn to the right 90 degrees. The “dot notation” is ...Python String Methods: Overview. Python String Methods that Return a Modified Version of a String. Python String Methods for Joining and Splitting Strings. …Jan 11, 2024 ... Python Strings Tutorial | All String Functions in Python One Shot | Python Course For Beginners Time Stamps ⌚ 00:01:06 - String ...We would like to show you a description here but the site won’t allow us.Lists are one of 4 built-in data types in Python used to store collections of data, the other 3 are Tuple, Set, and Dictionary, all with different qualities and usage. Lists are created using square brackets: Example. Create a List: thislist = ["apple", "banana", "cherry"] print (thislist) Try it Yourself ».VIN stands for vehicle identification number, and it’s a 17-character string of letters and numbers that tell you about the vehicle’s specifications and its manufacturing history. ...The replace() method does not change the string it is called on. The replace() method returns a new string. The replace() method replaces only the first match. If you want to replace all matches, use a regular expression with the /g flag set. See examples below. Python string is the collection of the characters surrounded by single quotes, double quotes, or triple quotes. The computer does not understand the characters; internally, it stores manipulated character as the combination of the 0's and 1's. Each character is encoded in the ASCII or Unicode character. So we can say that Python strings are ... Python String Methods. Python string class has a set of methods, which we can use on String objects. In this tutorial, we shall go through each of the method, what it does, … In this video course, you’ll learn how to: Manipulate strings with string methods. Work with user input. Deal with strings of numbers. Format strings for printing. This video course is part of the Python Basics series, which accompanies Python Basics: A Practical Introduction to Python 3. You can also check out the other Python Basics courses. Python strings have a lot of functionality that you can leverage in your scripts. This includes common text operations like searching and replacing text, removing …Jun 15, 2012 at 13:45. Add a comment. 3. You can use str.split ( [sep [, maxsplit]]) Return a list of the words in the string, using sep as the delimiter string. If maxsplit is given, at most maxsplit splits are done (thus, the list will have at most maxsplit+1 elements).Strings in Python are represented using the built-in str type/class. This class defines methods which can be used to manipulate or extract information from string instances. …Add a comment. 9. def kill_char(string, n): # n = position of which character you want to remove. begin = string[:n] # from beginning to n (n not included) end = string[n+1:] # n+1 through end of string. return begin + end. print kill_char("EXAMPLE", 3) # "M" removed. I have seen this somewhere here. Share.Reverse string in Python using stack. An empty stack is created. One by one character of the string is pushed to the stack. One by one all characters from the stack are popped and put back to a string. Time complexity: O(n) Auxiliary Space: O(n) Implementation:In Python Basics: Strings and String Methods, you used strings for text data in Python. You also learned how to manipulate strings with string methods. For example, you changed strings from lowercase to uppercase, removed whitespace from the beginning or end of a string, and replaced parts of a string with different text. In this video course, you’ll practice: Manipulating …. The file name is the module name with the suffix .py apStrings are an essential data type in Python that Mar 22, 2021 ... TABLE OF CONTENTS - Python String Methods 07:20 - capitalize() 08:48 - casefold() 10:12 - center() 11:55 - count() 14:45 - encode() 17:36 ... You don’t have to dig a big hole in your yard to Feb 19, 2010 · From the Python manual. string.find(s, sub[, start[, end]]) Return the lowest index in s where the substring sub is found such that sub is wholly contained in s[start:end]. Return -1 on failure. Defaults for start and end and interpretation of negative values is the same as for slices. And: string.index(s, sub[, start[, end]]) The `lower ()` method is a string method in Python. When a...
Continue Reading