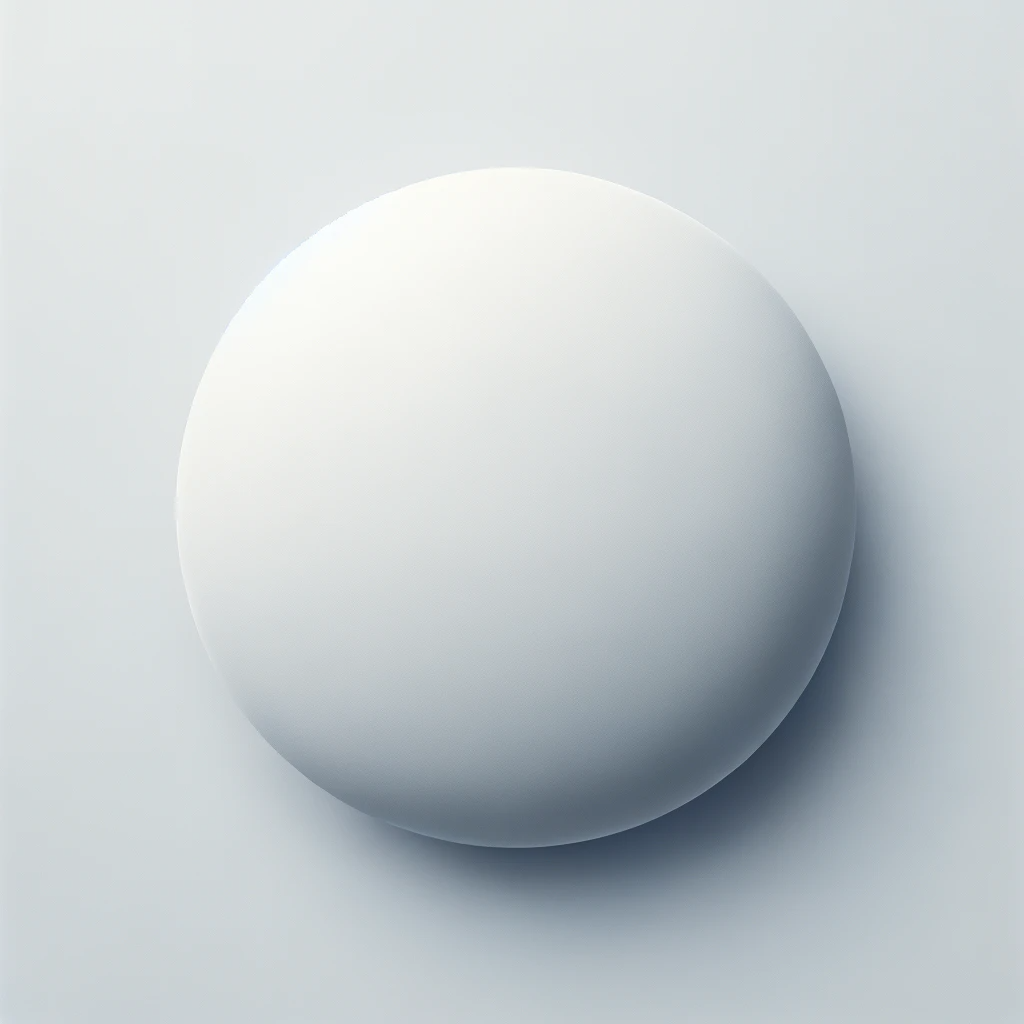
Using os.listdir () method to get the list of files. os.listdir () method gets the list of all files and directories in a specified directory. By default, it is the current directory. Beyond the first level of folders, os.listdir () does not return any files or …inline=True, we configured the checklist options to be displayed horizontally. This property is a shorthand for setting it on the labelStyle property and is available from Dash 2.1. The same can be done with labelStyle={'display': 'inline-block'} in earlier versions of Dash.. Options and Value. The options and value properties are the …How to Convert a List to an Array in Python. To convert a list to an array in Python, you can use the array module that comes with Python's standard library. The array module provides a way to create arrays of various types, such as signed integers, floating-point numbers, and even characters.This will create a Python list with three elements and assign it to the variable my_list . The square brackets [ and ] in this case mean "create a list" and the ...Create a List in Python. Here are the three different ways to get a list in Python with examples: using square brackets. list () type casting. list comprehension. …Learn how to create a list in Python using a template and examples. Also, learn how to access items in a list by index, range, or arithmetic operations.According to the Python Documentation: If no argument is given, the constructor creates a new empty list, []. 💡 Tip: This creates a new list object in memory and since we didn't pass any arguments to list (), an empty list will be created. For example: num = list () This empty list will have length 0, as you can see right here:The files required in to-do list project are: tasks.txt – The text file where all our tasks will be stored. main.py – The python script file. Here are the steps you will need to execute to build this python project: Importing all the necessary libraries. Initializing the window and placing all the components in it.Creating Lists in Python. We create lists in Python using square brackets [ ]. To create a list, enclose elements or values in square brackets separated by ...To create and write into a csv file. The below example demonstrate creating and writing a csv file. to make a dynamic file writer we need to import a package import csv, then need to create an instance of the file with file reference Ex:- with open("D:\sample.csv","w",newline="") as file_writerYou're technically trying to index an uninitialized array. You have to first initialize the outer list with lists before adding items; Python calls this "list comprehension". # Creates a list containing 5 lists, each of 8 items, all set to 0 w, h = 8, 5 Matrix = [[0 for x in range(w)] for y in range(h)] #You can now add items to …I'm going through a whole bunch of tuples with a many-to-many correlation, and I want to make a dictionary where each b of (a,b) has a list of all the a's that correspond to a b. It seems awkward to test for a list at key b in the dictionary, then look for an a, then append a if it's not already there, every single time through the tuple digesting loop; but I haven't … Learn how to create lists in Python using square brackets, and how to access, modify, and delete list items. Lists are ordered, changeable, and allow duplicate values. Objective: Create a list with data and make use of that list by passing it to a function. The vertices of a star centered the middle of a small drawing area are ...Learn how to create lists in Python using square brackets, and how to access, modify, and delete list items. Lists are ordered, changeable, and allow duplicate values.Creating a list of integers in Python. Ask Question Asked 2 years, 7 months ago. Modified 7 months ago. Viewed 11k times -3 Is it possible to create a list of integers with a single line of code, without using any third-party libraries? ... You can try this 2 situations to create a list: In this case, numbers without separation would be placed ...First you cannot slice a generator. Second this will not return the exact result, you probably meant splitted = column.split (). Third , the slice should actually be written splitted [::2] and splitted [1::2], Fourth may not work with all form of iterables, port your solution to use islice. – Abhijit.How to Create a List in Python. To create your first list in python we will program a small code that will create a list and output the list on the screen. Let's program . Program: #To create an empty list first list1= [] #Add items in the list list1 = ['Bike', 'Car', 2020, 1990] #Print list using print function print (list1)Creating a Python list. Length of a List. Accessing items of a List. Indexing. Negative Indexing. List Slicing. Iterating a List. Iterate along with an index number. … A Python list is a convenient way of storing information altogether rather than individually. As opposed to data types such as int, bool, float, str, a list is a compound data type where you can group values together. In Python, it would be inconvenient to create a new python variable for each data point you collected. Python offers the following list functions: sort (): Sorts the list in ascending order. type (list): It returns the class type of an object. append (): Adds a single element to a list. extend (): Adds multiple elements to a list. index (): Returns the first appearance of the specified value.Create your own server using Python, PHP, React.js, Node.js, Java, C#, etc. How To's. Large collection of code snippets for HTML, CSS and JavaScript. ... Copy a List. You cannot copy a list simply by typing list2 = list1, because: list2 will only be a reference to list1, ...Feb 16, 2023 · How to Create a List in Python. You can create a list in Python by separating the elements with commas and using square brackets []. Let's create an example list: myList = [3.5, 10, "code", [ 1, 2, 3], 8] From the example above, you can see that a list can contain several datatypes. In order to access these elements within a string, we use ... 1. String to List of Strings. When we need to convert a string to a list in Python containing the constituent strings of the parent string (previously separated by some separator like ‘,’ or space), we use this method to accomplish the task.. For example, say we have a string “Python is great”, and we want a list that would contain only the given …7 Answers. Sorted by: 3. Use a dict. mylists = {} x = 'abhishek'. mylists[x] = [] That way, in mylists you'll have all your lists. mylists [x] is the list with name x.To create a list of strings, first use square brackets [ and ] to create a list. Then place the list items inside the brackets separated by commas. Remember that strings must be surrounded by quotes. Also remember to use = to store the list in a variable. So we get something like this:These are just a few examples of how you can create a list in Python. Once you have created a list, you can access, modify, and perform various operations on the list using Python’s built-in list methods. Accessing data from Lists. In Python, you can access individual items or a range of items from a list using their index.The general syntax looks something like this: list_name.append(item) Let's break it down: list_name is the name you've given the list. .append () is the list method for adding an item to the end of list_name. item is the specified individual item you want to add. When using .append (), the original list gets modified.You can make use of glob. glob.glob(pathname, *.jpg, recursive=False) Return a possibly-empty list of path names that match pathname, which must be a string containing a path specification. pathname can be either absolute (like /usr/src/Python-1.5/Makefile) or relative (like ../../Tools//.gif), and can contain shell-style wildcards.. …Python. pal_gen = infinite_palindromes() for i in pal_gen: digits = len(str(i)) pal_gen.send(10 ** (digits)) With this code, you create the generator object and iterate through it. The program only yields a value once a palindrome is found. It uses len () to determine the number of digits in that palindrome.Create a list of floats in Python using map () Function. Another approach to create a list of floats is by using the map () function. This function applies a given function to each item of an iterable (e.g., list, tuple) and returns a new iterable (e.g., a list or a map object).I want to create a very longer list, but ,in python (i don't know in another one), the lists have a limit (24~30, i think), the question is this: Is it ...Create a List of Lists Using append () Function. In this example the code initializes an empty list called `list_of_lists` and appends three lists using append () …You probably want to create a list in your function, use the yield keyword, or use the built in list function.. def generateNumberList(num): myList = [] for i in range(num): myList.append(i) #Notice that your return the list you've created rather #than each individaul integer return myList print generateNumberList(10) def generateNumberList2(num): for i in range(10): …What is the first thing you’d do? Have the answer already? Yes, you'd probably write down a shopping list! Python also has a built-in data structure called List that’s very similar to your shopping list. This …Aug 14, 2023 ... You can create an empty list in Python using either square brackets [] or the list() function. An empty list can be used as a placeholder for ...A list is generated in Python programming by putting all of the items (elements) inside square brackets [], separated by commas. It can include an unlimited number of elements of various data types (integer, float, string, etc.). Python Lists can also be created using the built-in list () method. Example.Create your own server using Python, PHP, React.js, Node.js, Java, C#, etc. How To's. Large collection of code snippets for HTML, CSS and JavaScript. ... Copy a List. You cannot copy a list simply by typing list2 = list1, because: list2 will only be a reference to list1, ...A fixed-size list is a list that has a predefined number of elements and does not change in size. In Python, while lists are inherently dynamic, we can simulate ...all the top solutions work for the example of the question, but they don't answer the questions. They all use set, which is dependent on the types found in the list.e.g: d = dict();l = list();l.append (d);set(l) will lead to TypeError: unhashable type: 'dict.frozenset instead won't save you. Learn it the real pythonic way: …Here we have one condition. Example 1. Condition: only even numbers will be added to new_list. new_list = [expression for item in iterable if condition == True] new_list = [x for x in range(1, 10) if x % 2 == 0] > [2, 4, 6, 8] Example 2. Condition: only even numbers that are multiple of 3 will be added to new_list. In python, as far as I know, there are at least 3 to 4 ways to create and initialize lists of a given size: Simple loop with append:. my_list = [] for i in range(50): my_list.append(0) The list is : [1, 4, 5, 7, 8] Length of list using len() is : 5. Length of list using length_hint() is : 5. 4. Find the Length of a List Using sum () Function. Use iteration inside the sum and with each iteration adds one and at the end of the iteration, we get the total length of the list. Python3.List.append () method is used to add an element to the end of a list in Python or append a list in Python, modifying the list in place. For example: `my_list.append (5)` adds the element `5` to the end of the list `my_list`. Example: In this example, the In below code Python list append () adds a new element at … In python, as far as I know, there are at least 3 to 4 ways to create and initialize lists of a given size: Simple loop with append:. my_list = [] for i in range(50): my_list.append(0) Feb 25, 2022 · <class 'list'> <class 'list'> How to Access an Item within a list. You can access an item within a list in Python by referring to the item’s index. Each item within a list has an index number associated with that item (starting from zero). For example, recall that the template to create a list is: What is Linked List in Python. A linked list is a type of linear data structure similar to arrays. It is a collection of nodes that are linked with each other. A node contains two things first is data and second is a link that connects it with another node. Below is an example of a linked list with four nodes and each node …Understanding these differences is crucial when choosing the right data structure for your specific use case. Creating Lists. Python offers multiple ways to ...Numpy's r_ convenience function can also create evenly spaced lists with syntax np.r_[start:stop:steps]. If steps is a real number (ending on j ), then the end point is included, equivalent to np.linspace(start, stop, step, endpoint=1) , otherwise not.inline=True, we configured the checklist options to be displayed horizontally. This property is a shorthand for setting it on the labelStyle property and is available from Dash 2.1. The same can be done with labelStyle={'display': 'inline-block'} in earlier versions of Dash.. Options and Value. The options and value properties are the …Python provides a nicer way to do this kind of task by using the map() built-in function. The map() function iterates over all elements in a list (or a tuple), applies a function to each, and returns a new iterator of the new elements. The following shows the basic syntax of the map() function: iterator = map(fn, list) Code language: Python ...To generate a list in Python, add a generator expression to the code using the following syntax: generator = ( expression for element in iterable if condition ). For example: my_gen = ( x**2 for x in range (10) if x%2 == 0 ). This differs from the Python list comprehension syntax by using parentheses instead of square brackets.You can make a shorter list in Python by writing the list elements separated by a comma between square brackets. Running the code squares = [1, 4, 9, 16, ...Here we have one condition. Example 1. Condition: only even numbers will be added to new_list. new_list = [expression for item in iterable if condition == True] new_list = [x for x in range(1, 10) if x % 2 == 0] > [2, 4, 6, 8] Example 2. Condition: only even numbers that are multiple of 3 will be added to new_list.depending on how you use it, it may be better to omit the list part (or for python 2: use xrange). e.g if you iterate once through every member of the range in a for loop. Share. Improve this answer. ... How do I create a list with the number I want in a loop? 0. how can ı make a list consist of integers from given list. 0.depending on how you use it, it may be better to omit the list part (or for python 2: use xrange). e.g if you iterate once through every member of the range in a for loop. Share. Improve this answer. ... How do I create a list with the number I want in a loop? 0. how can ı make a list consist of integers from given list. 0.I have a list of variable length and want to create a checkbox (with python TKinter) for each entry in the list (each entry corresponds to a machine which should be turned on or off with the checkbox -> change the value in the dictionary). print enable {'ID1050': 0, 'ID1106': 0, 'ID1104': 0, 'ID1102': 0} (example, can be any length)The syntax for the “not equal” operator is != in the Python programming language. This operator is most often used in the test condition of an “if” or “while” statement. The test c...Create a List with list comprehension in Python. List comprehension offers a shorter syntax when we want to create a new list based on the values of an existing list. List comprehension is a sublime way to define and build lists with the help of existing lists. In comparison to normal functions and … Lists and tuples are arguably Python’s most versatile, useful data types. You will find them in virtually every nontrivial Python program. Here’s what you’ll learn in this tutorial: You’ll cover the important characteristics of lists and tuples. You’ll learn how to define them and how to manipulate them. Mar 7, 2024 ... In this tutorial, we will explore ways to Create, Access, Slice, Add, Delete Elements to a Python List along with simple examples.Python has become one of the most widely used programming languages in the world, and for good reason. It is versatile, easy to learn, and has a vast array of libraries and framewo...#splits string according to delimeters ''' Let's make a function that can split a string into list according the given delimeters. example data: cat;dog:greff,snake/ example delimeters: ,;- /|: ''' def string_to_splitted_array(data,delimeters): #result list res = [] # we will add chars …Closed yesterday. I am running some code to deal with data via Pandas. I have imported the .CSV and iterated over df.columns to create a list of years to use for …This approach creates a list of objects by iterating over a range of numbers and creating a new object for each iteration. The objects are then appended to a list using a list comprehension. Python3. class geeks: def __init__ (self, name, roll): self.name = name. self.roll = roll.2. Create an Array of Strings using Python List. There is no built-in array data structure in Python specifically for storing strings. However, you can use a list to …Use the * Operator to Create a List of Zeros in Python. If we multiple a list with a number n using the * operator, then a new list is returned, which is n times the original list. Using this method, we can easily create a list containing zeros of some specified length, as shown below. lst = [0] * 10 print(lst)2. Create an Array of Strings using Python List. There is no built-in array data structure in Python specifically for storing strings. However, you can use a list to …If we compare the runtimes, among random list generators, random.choices is the fastest no matter the size of the list to be created. However, for larger lists/arrays, numpy options are much faster. So for example, if you're creating a random list/array to assign to a pandas DataFrame column, then using …Create a List of Lists Using append () Function. In this example the code initializes an empty list called `list_of_lists` and appends three lists using append () function to it, forming a 2D list. The resulting structure is then printed using the `print` statement. Python.Dec 16, 2011 · There is a gotcha though, both itertools.repeat and [0] * n will create lists whose elements refer to same id. This is not a problem with immutable objects like integers or strings but if you try to create list of mutable objects like a list of lists ([[]] * n) then all the elements will refer to the same object. Modern society is built on the use of computers, and programming languages are what make any computer tick. One such language is Python. It’s a high-level, open-source and general-...This means that Python doesn’t create the individual elements of a range when you create the ... Lists are eagerly constructed, so that all elements in the list are present in memory immediately when the list is created. That’s why you converted ranges into lists to inspect the individual elements earlier: Python >>> range (1, …What is Python Nested List? A list can contain any sort object, even another list (sublist), which in turn can contain sublists themselves, and so on. This is known as nested list.. You can use them to arrange data into hierarchical structures. Create a Nested List. A nested list is created by placing a comma-separated sequence of …To generate a list in Python, add a generator expression to the code using the following syntax: generator = ( expression for element in iterable if condition ). For example: my_gen = ( x**2 for x in range (10) if x%2 == 0 ). This differs from the Python list comprehension syntax by using parentheses instead of square brackets.Step 1: Define the Node Structure. First, we need to define the structure of a node in the linked list. Each node will contain some data and a pointer to the next node. …Because you're appending empty_list at each iteration, you're actually creating a structure where all the elements of imp_list are aliases of each other. E.g., if you do imp_list[1].append(4), you will find that imp_list[0] now also has that extra element. So, instead, you should do imp_list.append([]) and make each …When you run runRounds you need to pass in the lists as parameters because you aren't creating any in the function. At the end of it, you need to return the lists, so they can be accessed later. For runTimes you need to pass in the numRounds variable you created earlier and the finalList because you are creating firstList in the …Let’s discuss a few approaches to Creating a list of numbers with a given range in Python. Naive Approach using a loop. A naive method to create a list within a given range is to first create an empty list and append the successor of each integer in every iteration of for loop.List comprehension offers a shorter syntax when you want to create a new list based on the values of an existing list. Example: Based on a list of fruits, you want a new list, containing only the fruits with the letter "a" in the name. Without list comprehension you will have to write a for statement with a conditional test inside:Closed yesterday. I am running some code to deal with data via Pandas. I have imported the .CSV and iterated over df.columns to create a list of years to use for …Are you an intermediate programmer looking to enhance your skills in Python? Look no further. In today’s fast-paced world, staying ahead of the curve is crucial, and one way to do ...Python offers the following list functions: sort (): Sorts the list in ascending order. type (list): It returns the class type of an object. append (): Adds a single element to a list. extend (): Adds multiple elements to a list. index (): Returns the first appearance of the specified value.. Sep 20, 2010 · Explore Teams Create a freeHow to Create a List in Python. To create your first list in python Dec 16, 2011 · There is a gotcha though, both itertools.repeat and [0] * n will create lists whose elements refer to same id. This is not a problem with immutable objects like integers or strings but if you try to create list of mutable objects like a list of lists ([[]] * n) then all the elements will refer to the same object. This means that Python doesn’t create the individual elements of a Python is a powerful and versatile programming language that has gained immense popularity in recent years. Known for its simplicity and readability, Python has become a go-to choi... Python Generator Expression. In Python, a generator expression ...
Continue Reading